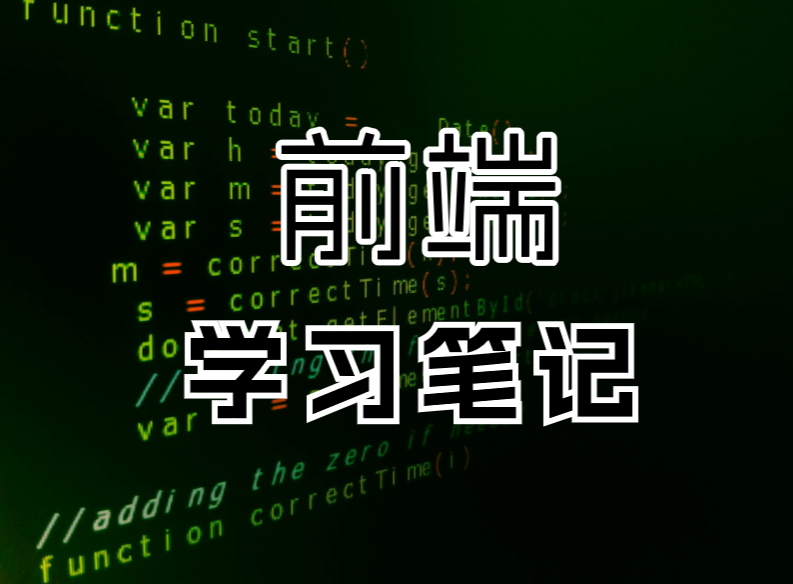
商米PDA扫码-UNIAPP连接
背景
提高扫码效率
理论
PDA扫码通过广播的方式推送给APP,更具PDA的提供的广播信道
filter.addAction("com.sunmi.scanner.ACTION_DATA_CODE_RECEIVED");
和 intent.getByteArrayExtra("source_byte")
设置监听的广播和key
实现代码
padjs
// @/utils/pda.js
const timeout = 5 * 1000; //扫码超时时间
const main = plus.android.runtimeMainActivity();
// 全局只允许一个地方获取,存储最新的回调函数,获取完立即销毁
let scanCallback = null;
//存储订阅,每个页面可以单独订阅,优先级低于scanCallback
const subscriptions = [];
export const byteArrayToString = (array) => {
return String.fromCharCode.apply(String, array);
};
const sleep = (ms) => {
return new Promise((resolve) => setTimeout(resolve, ms));
};
export const registerReceiver = () => {
//获取Android意图过滤类
const IntentFilter = plus.android.importClass("android.content.IntentFilter");
//实例化意图过滤
const filter = new IntentFilter();
//获取扫码成功的意图广播,
filter.addAction("com.sunmi.scanner.ACTION_DATA_CODE_RECEIVED");
const receiver = plus.android.implements(
"io.dcloud.feature.internal.reflect.BroadcastReceiver", {
onReceive: function(context, intent) {
plus.android.importClass(intent);
console.log('intent', context, intent);
const dataByte = intent.getByteArrayExtra("source_byte");
console.log('dataByte', dataByte);
const code = byteArrayToString(dataByte);
console.log("receiver:" + code);
if (scanCallback) {
scanCallback(code);
} else {
subscriptions.forEach((cb) => cb(code));
}
},
}
);
main.registerReceiver(receiver, filter);
};
export const openScan = () => {
//获取Android意图类
let Intent = plus.android.importClass("android.content.Intent");
//实例化意图
let intent = new Intent();
//定义意图,由厂商提供
intent.setAction("com.sunmi.scanner.ACTION_DATA_CODE_RECEIVED");
//广播这个意图
main.sendBroadcast(intent);
};
export const scanCode = async () => {
uni.showLoading({
title: "扫描中",
mask: true
});
const getCode = () => {
return new Promise((resolve) => {
scanCallback = (code) => {
resolve(code);
};
});
};
openScan();
const res = await Promise.race([getCode(), sleep(timeout)]);
scanCallback = null;
uni.hideLoading();
if (!res) {
uni.showToast({
icon: "none",
title: "扫描失败",
});
throw new Error("扫描失败");
}
return res;
};
export const addSubscription = (callback) => {
if (callback) {
subscriptions.push(callback);
}
};
export const removeSubscription = (callback) => {
const index = subscriptions.findIndex((v) => v === callback);
if (index > -1) {
subscriptions.splice(index, 1);
}
};
注册扫码事件
// appjs
import {registerReceiver} from '@/utils/pda.js'
页面内使用
import { addSubscription, removeSubscription } from "@/utils/scan";
export default {
onShow() {
if (this.onReceiveCode) {
addSubscription(this.onReceiveCode);
}
},
onHide() {
if (this.onReceiveCode) {
removeSubscription(this.onReceiveCode);
}
},
beforeDestroy() {
if (this.onReceiveCode) {
removeSubscription(this.onReceiveCode);
}
}
}
其中
onReceiveCode(code) {
// 自己的业务
}
- 感谢你赐予我前进的力量
赞赏者名单
因为你们的支持让我意识到写文章的价值🙏
评论
匿名评论
隐私政策
你无需删除空行,直接评论以获取最佳展示效果